Break:
- A break statement can be used to terminate or to come out from the loop or conditional statement unconditionally.
- It can be used in switch statement to break and come out from the switch statement after each case expression.
- Whenever, break statement is encounter within the program then it will break the current loop or block.
- A break statement is normally used with if statement.
- When certain condition becomes true to terminate the loop then break statement can be used.
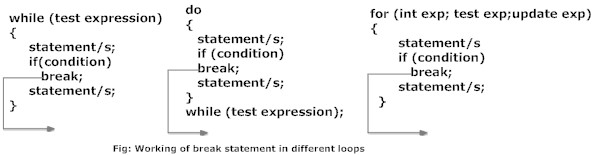
- In the following program demonstrates the use of break statement. Loop will be terminated as soon as the counter value becomes greater than 5.
/* program to demonstrate the use of continue */
#include "stdio.h"
#include "conio.h"
void main()
{
int i;
clrscr();
for( i = 1; i <= 10 ; i++ )
{
if (i > 5)
break; // terminate loop
printf("\n %d ", i) ;
}
getch();
}
Continue:
- A continue statement can be used into the loop when we want to skip some statement to be executed and continue the execution of above statement based on some specific condition.
- Similar to break statement, continue is also used with if statement.
- When compiler encounters continue, statements after continue are skip and control transfers to the statement above continue.

- The following example uses the continue statement to print upper and lower a to z alphabets
/* program to print upper and lower a to z alphabets using continue */
#include "stdio.h"
#include "conio.h"
void main()
{
int i;
clrscr();
for ( i = 65 ; i<=122; i++ ) // loop through ASCII value for a to z
{
if(i >= 91 && i <= 96)
continue ; // skip unnecessary special characters.
printf("| %c " , i ) ; // print character equivalent for ASCII value.
}
getch();
}
Exit:
- An exit statement is used to terminate the current execution flow.
- It is an in-built system function it is available in process h. header file.
- As soon as exit statement is found, it will terminate the program.
- It has single argument of zero. For example: exit(0);
- the following program demonstrates the use of both break and exit statements.
/* Program to demonstrate the use of break & exit statement for basic calculation
#include<stdio.h>
#include<conio.h>
void main()
{
float x, y, z;
int ch;
clrscr();
printf("\n1.Addition\n2.Subtraction\n3.Multiplication\4.Division\n5.Exit\n");
printf("\nEnter your choice :");
scanf("%d", &ch);
printf("\nEnter X and Y :");
scanf("%f %f", &x, &y);
switch(ch)
{
case 1:
z = x + y;
break;
case 2:
z = x - y;
break;
case 3:
z = x * y;
break;
case 4:
z = x / y;
break;
case 5:
printf("\n Good Bye!");
exit(0);
default:
printf("\n Invalid choice! Please try again!");
}
printf("\n Answer is %.2f", z);
getch();
}